
petFeeder plugin
Moderator: Moderators
-
- Noob
- Posts: 3
- Joined: 03 Sep 2008, 22:56
- Noob?: Yes
- Location: Malaysia
Re: petFeeder plugin
is link working "http://bibian.ath.cx/openkore/viewtopic.php?t=4647" ? 

-
- Human
- Posts: 39
- Joined: 15 Apr 2008, 18:58
- Noob?: Yes
Re: petFeeder plugin
Nope.hasyim1987 wrote:is link working "http://bibian.ath.cx/openkore/viewtopic.php?t=4647" ?
-
- Super Moderators
- Posts: 801
- Joined: 06 May 2008, 12:47
- Noob?: No
Re: petFeeder plugin
updated version:
test please
Code: Select all
# petFeeder v1.6
# -by punkpudding
package petFeeder;
use strict;
use Time::HiRes qw(time);
use Globals;
use Plugins;
use Log qw(debug message warning error);
use Utils;
use Commands;
use Network;
use Network::Send;
# not the official enum
use constant {
PET_INFO => 0x0,
PET_FEED => 0x1,
PET_PERFORMANCE => 0x2,
PET_TOEGG => 0x3,
PET_UNEQUIP => 0x4,
};
Plugins::register('petFeeder', 'petFeeder by punkpudding', \&onUnload, \&onReload);
my $hookCommandPost = Plugins::addHook('Command_post', \&onCommandPost);
my $hookAIpre = Plugins::addHook('AI_pre', \&onAIpre);
my $hookParseMsgPre = Plugins::addHook('parseMsg/pre', \&onParseMsgPre);
message "Pet feeding plugin loaded\n", "success";
my %pet;
my $hunger = 72;
my $performanceRate = $config{petPerformanceRate};
sub onUnload {
Plugins::delHook('parseMsg/pre', $hookParseMsgPre);
Plugins::delHook('AI_pre', $hookAIpre);
Plugins::delHook('Command_post', $hookCommandPost);
message "Pet feeding plugin unloaded\n", "success";
}
sub onReload {
&onUnload;
}
sub onCommandPost {
my (undef, $args) = @_;
my ($cmd, $subcmd) = split(' ', $args->{input}, 2);
if ($cmd eq "pet" && $config{petFeeder}) {
if ($subcmd eq "s" || $subcmd eq "status") {
message "Pet status: hungry=$pet{hungry} intimacy=$pet{friendly}\n";
} elsif ($subcmd eq "f" || $subcmd eq "feed") {
if ($pet{hungry} <= 72) {
message "Feeding your pet. [current intimacy : $pet{friendly}]\n";
$messageSender->sendPetMenu(PET_FEED);
$messageSender->sendPetMenu(PET_INFO);
} elsif ($pet{hungry} > 72) {
message "Your pet's not yet hungry. Feeding him now will lower intimacy.\n";
}
} elsif ($subcmd eq "p" || $subcmd eq "performance") {
message "Playing your pet.\n";
$messageSender->sendPetMenu(PET_PERFORMANCE);
} elsif ($subcmd eq "r" || $subcmd eq "return") {
message "Returning pet to egg status.\n";
$messageSender->sendPetMenu(PET_TOEGG);
} elsif ($subcmd eq "u" || $subcmd eq "unequip") {
message "Unequipping pet accessory.\n";
$messageSender->sendPetMenu(PET_UNEQUIP);
} else {
error "Syntax Error in function 'pet' (pet management)\n" .
"Usage: pet < s[tatus] | f[eed] | p[erformance] | r[eturn] | u[nequip] >\n";
}
$args->{return} = 1;
}
}
sub onAIpre {
return unless ($main::conState == 5);
if ($config{petFeeder}) {
# request status
if (timeOut($pet{lastStatusInfo}, 30)) {
if (timeOut($pet{lastStatusRequest}, 10)) {
$pet{lastStatusRequest} = time;
$messageSender->sendPetMenu(PET_INFO);
if ($pet{friendly} < 990) {
$hunger = 72;
} elsif ($pet{friendly} >=990) {
$hunger = 30;
}
}
}
# pet performance
if ((defined($config{petPerformanceRate}) && timeOut($pet{lastPerformance}, $performanceRate)) &&
((!$config{petPerformance_onAction}) || ($config{petPerformance_onAction} && existsInList($config{petPerformance_notOnAction}, AI::action()))) &&
((!$config{petPerformance_notOnAction}) || ($config{petPerformance_notOnAction} && !existsInList($config{petPerformance_notOnAction}, AI::action())))) {
$messageSender->sendPetMenu(PET_PERFORMANCE);
message "Auto-playing your pet\n";
$performanceRate = $config{petPerformanceRate} if !defined($performanceRate);
$performanceRate = $config{petPerformanceRate} + int(rand $config{petPerformanceRateSeed}) if $config{petPerformanceRateSeed};
$pet{lastPerformance} = time;
}
}
}
sub onParseMsgPre {
my (undef, $args) = @_;
my $switch = $args->{switch};
my $msg = $args->{msg};
# process status
if ($switch eq "01A2" && $config{petFeeder}) {
$pet{name} = substr($msg, 2, 24) =~ /([\s\S]*?)\000/;
$pet{nameflag} = unpack("C1", substr($msg, 26, 1));
$pet{level} = unpack("S1", substr($msg, 27, 2));
$pet{hungry} = unpack("S1", substr($msg, 29, 2));
$pet{friendly} = unpack("S1", substr($msg, 31, 2));
$pet{accessory} = unpack("S1", substr($msg, 33, 2));
$pet{lastStatusInfo} = time;
debug "Pet status: level=$pet{level} hungry=$pet{hungry} intimacy=$pet{friendly}\n";
if ($config{petSmartFeed}) {
if ($pet{hungry} < $hunger && timeOut($pet{lastFeed}, 5) && $pet{friendly} > 0) {
$pet{lastFeed} = time;
$messageSender->sendPetMenu(PET_FEED);
$messageSender->sendPetMenu(PET_INFO);
message "Auto-feeding your pet. [current intimacy : $pet{friendly}]\n";
}
if ($pet{hungry} <= $config{petMinHunger} || $pet{hungry} <= 15) {
$messageSender->sendPetMenu(PET_TOEGG);
message "Critical hunger level reached. Pet returned to egg status.\n";
}
} else {
if ($pet{hungry} < $config{petFeedRate} && timeOut($pet{lastFeed}, 5) && $pet{friendly} > 0) {
$pet{lastFeed} = time;
$messageSender->sendPetMenu(PET_FEED);
$messageSender->sendPetMenu(PET_INFO);
message "Auto-feeding your pet. [current intimacy : $pet{friendly}]\n";
}
if ($pet{hungry} <= $config{petMinHunger} || $pet{hungry} <= 15) {
$messageSender->sendPetMenu(PET_TOEGG);
message "Critical hunger level reached. Pet returned to egg status.\n";
}
}
}
}
return 1;
One ST0 to rule them all? One PE viewer to find them!
One ST_kRO to bring them all and in the darkness bind them...
Mount Doom awaits us, fellowship of OpenKore!
One ST_kRO to bring them all and in the darkness bind them...
Mount Doom awaits us, fellowship of OpenKore!
-
- Noob
- Posts: 3
- Joined: 03 Sep 2008, 22:56
- Noob?: Yes
- Location: Malaysia
Re: petFeeder plugin
this code need put which folders., the link dead.... 

-
- Moderators
- Posts: 403
- Joined: 25 Aug 2008, 14:56
- Noob?: No
- Location: CyberOne Building , Eastwood
Re: petFeeder plugin
hasyim1987 wrote:this code need put which folders., the link dead....
http://wiki.openkore.com/index.php/Category:Plugins
“The moon shines to both guilty and innocent alike..”
The Openkore Manual---Global Forum Rules--The Template
The Openkore Manual---Global Forum Rules--The Template
-
- Noob
- Posts: 10
- Joined: 30 Aug 2010, 11:01
- Noob?: No
- Location: KL
Re: petFeeder plugin
Working fine. But have a bug where it's conflict with homunculus pet as it overwrite homun feed.
-
- Noob
- Posts: 10
- Joined: 30 Aug 2010, 11:01
- Noob?: No
- Location: KL
Re: petFeeder plugin
There is bug in it. It will actually replace the homun feed. So if you want to active this, better keep your homun in rest. Can't have both pet and homun active in a time or else the bot will not feed the homun.
-
- Noob
- Posts: 4
- Joined: 17 Dec 2010, 08:19
- Noob?: Yes
Re: petFeeder plugin
Can you specify the hunger rate?
Hungry is what value??
Neutral is what value??
Stuffed is what value??
BTW.. my Isis keep feeling Awkward T.T
Hungry is what value??
Neutral is what value??
Stuffed is what value??
BTW.. my Isis keep feeling Awkward T.T
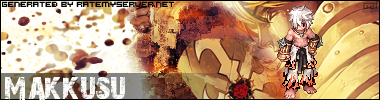
-
- Noob
- Posts: 4
- Joined: 17 Dec 2010, 08:19
- Noob?: Yes
Re: petFeeder plugin
I forgot to set my petfeeder to 1 and it did not return my pet to egg and lost my pet.. so is tat mean i need to set petfeeder to 1 in order to have all the function to work? BTW is there any command or bot to search for lost pet itself?
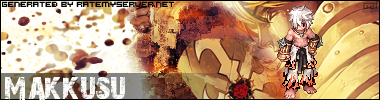
-
- Noob
- Posts: 1
- Joined: 10 Jun 2009, 02:02
- Noob?: Yes
Re: petFeeder plugin
HELP me!!!!! i use this updated version but my bot feed my pet twice...
so the intimacy down by -100 T_T then my pet got away T_T.... i check the plugin codes and there is no eror that call PET_FEED twice,
anyone know what might be the problem?
so the intimacy down by -100 T_T then my pet got away T_T.... i check the plugin codes and there is no eror that call PET_FEED twice,
anyone know what might be the problem?